Raspberry Pi Camera
Enable Camera
On Raspberry Pi OS-Desktop:
- access the start menu
Preferences->Raspberry Pi Configuration
On the command line:
sudo raspi-config
- enable/disable the camera in interface options
Verify Camera
vcgencmd
allows us to query the VideoCore GPU - we can use this to verify our camera has been detected:
vcgencmd get_camera # supported=1 detected=1 when successful
If this is unsuccessful, the PiCam cable may not be connected properly.
Take a Photo
raspistill -o image.jpg
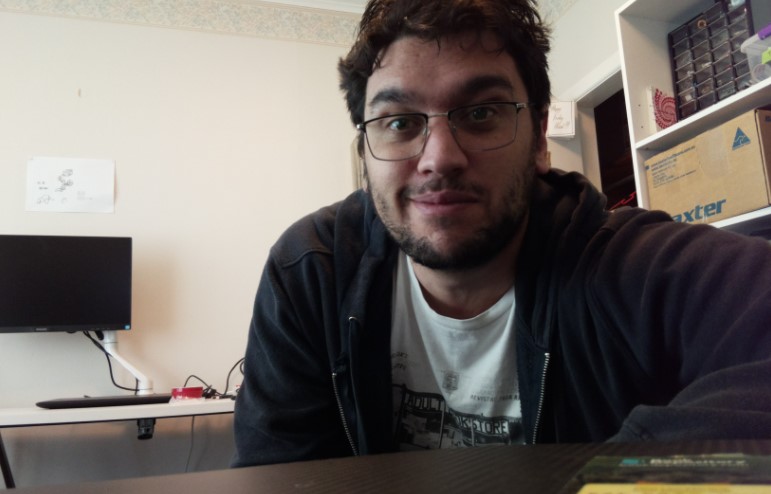
Run raspistill --help
to see all of the additional options and filtering available.
Another tool raspiyuv
generates uncompressed images which can be useful for computer vision.
Take a Video
raspivid -o vid.h264 -t 10000
The -t
flag sets how long to record in milliseconds
- default is
5000
milliseconds - set to
0
to record until cancelled
Streaming
Assuming we have a raspberry pi on 192.168.1.123
, and we would like to stream on port 5000
:
raspivid -t 0 -w 320 -h 240 --inline --listen -o tcp://0.0.0.0:5000
On client side:
vlc tcp/h264://192.168.1.123:5000
I have found that gstreamer has significantly lower latency than using raspivid
directly. See GStreamer RPi Streaming.
Python pycamera Library
Install the pycamera library on Raspberry Pi OS:
sudo apt update
sudo apt install python3-pycamera
Capturing an Image
from picamera import PiCamera
camera = PiCamera()
camera.capture('image.jpg')
Recording a Video
from picamera import PiCamera
camera = PiCamera()
camera.start_recording('video.h264')
camera.wait_recording(5)
camera.stop_recording()
See the Basic Recipies Documentation for more.